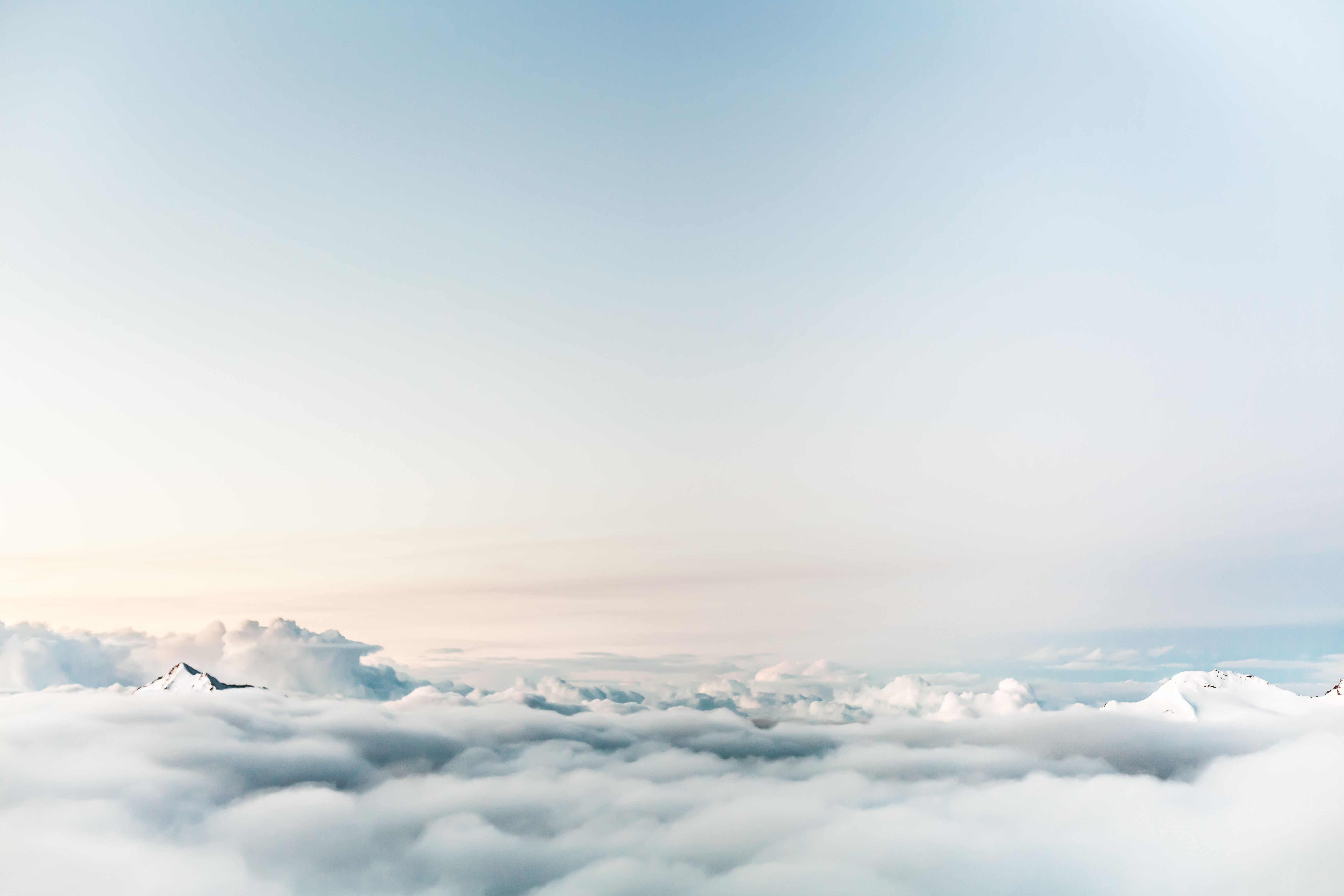
JavaScript basics
Table of Contents
What JavaScript is
- A scripting language originally designed for web browsers
- Object-oriented, however prototype-based and not class-based
- Supporting first-class functions
- A multi-paradigm language, supports imperative, object-oriented and functional programming style
- Untyped
- Moving from an interpreted language to just-in-time compilation
- Nowadays used for server-side programming too
- Relying on a host/run-time environment (web browser, Node.js)
JavaScript and the Browser
- The browser is the most common host environment
- It provides a graphical user interface (via DOM)
<!doctype html>
<html>
<head>
<title>JavaScript</title>
</head>
<body>
<script>
// Output to the console
console.log("Hello world");
</script>
</body>
</html>
Node.js
- Node.js is a server-side host environment
- Initially conceived to facilitate asynchronous I/O
let x = Math.floor((Math.random() * 10) + 1);
console.log("Your random number is: " + x);
this can be executed with:
$ node example.js
Your random number is: 42
Variable Declaration and Value Assignment
- Variables are declared using the let keyword or const if no reassignment is needed
- The old var keyword shouldn’t be used anymore
Tip: always use const. Only need let if you really need to change the variable later on
// Declare a variable
let x;
console.log(x); // >> undefined
// Assign a value to a variable
x = 42;
console.log(x); // >> 42
// Declare and assign in one stroke
let y = 2;
// Variables declared using ’let’ can be reassigned
y = 1;
// Variables declared using ’const’ cannot be reassigned
const z = 99;
z = 16; // ERROR
JavaScript is untyped
So JavaScript has no Types? Well it has, but not before runtime. JavaScript is dynamically typed, i.e., the type of a variable is determined at runtime and may change during program execution.
let x = 12;
console.log(typeof x); // >> number
// Values of different types can be assigned to a variable
x = "Hello World";
console.log(typeof x); // >> string
// Automatic type conversion
let y = 12+"10";
console.log(typeof y); // >> string ('1210')
y = 12-"10";
console.log(typeof y); // >> number (2)
// Explicit type conversion with Number(), String() and Boolean()
let z = 12+Number("10");
console.log(typeof z); // >> number
console.log(z); // >> 22
Block Scope
- Variables declared with let and const are block-scoped
- Before ES6, JavaScript had no block scope and hence variables declared with var are not block-scoped
{
let x = 12;
const y = 13;
}
// x and y cannot be used here
{
var z = 14;
}
console.log(z); // >> 14
Equality and Identicalness
- == and != compare only values (equality)
- === and !== compare values and types (identicalness)
const a = 7;
const b = "7";
// a and b are equivalent...
console.log(a == b); // >> true
// ... but not identical (number vs. string)
console.log(a === b); // >> false
console.log(a === parseInt(b)); // >> true
const x = 0;
const y = false;
// x and y are equivalent...
console.log(x == y); // >> true
// ...but not identical (number vs. boolean)
console.log(x === y); // >> false
Strict mode
The "use strict"
directive was new in ECMAScript version 5. It is not a statement, but a literal expression, ignored by earlier versions of JavaScript. The purpose of "use strict"
is to indicate that the code should be executed in "strict mode". With strict mode, you can not, for example, use undeclared variables. All modern browsers support "use strict". The numbers in the table specify the first browser version that fully supports the directive. You can use strict mode in all your programs. It helps you to write cleaner code, like preventing you from using undeclared variables.